Multi - threading is the ability to execute multiple different paths of code at the same time. Java program normally only use one thread.
Ways of creating a new thread
Next to the main class, create a new class (any name you like).
A class extends the thread class.
At the end of the new class add extends Thread.
MultiThread.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34public class MultiThread extends Thread
{
private int threadNum;
public MultiThread(int threadNum)
{
this.threadNum = threadNum;
}
public void run()
{
for (int i = 0; i <= 5; i++)
{
System.out.println(i + " from thread " + threadNum);
/* test of multi-thread
if (threadNum == 3)
{
throw new RuntimeException();
}
*/
try
{
Thread.sleep(1000);
}
catch (InterruptedException e)
{
e.printStackTrace();
}
}
}
}
Main.java
1
2
3
4
5
6
7
8
9
10
11
12
13public class Main
{
public static void main(String[] args)
{
for (int i = 0; i <= 3; i++)
{
MultiThread thread = new MultiThread(i);
thread.start();
}
// Multi-thread test
//throw new RuntimeException();
}
}
A class implements the Runnable interface.
At the end of the new class add implements Runnable.
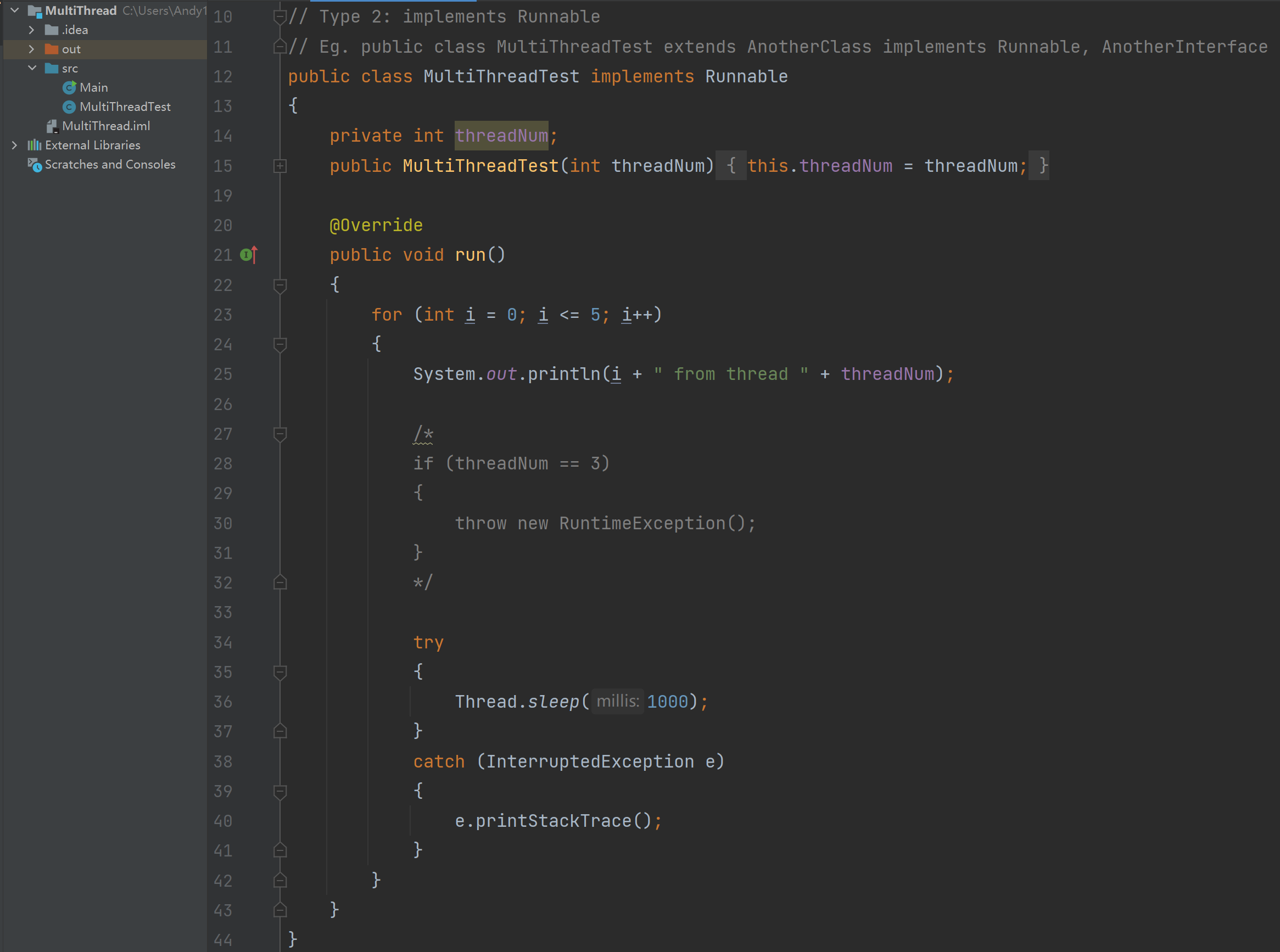
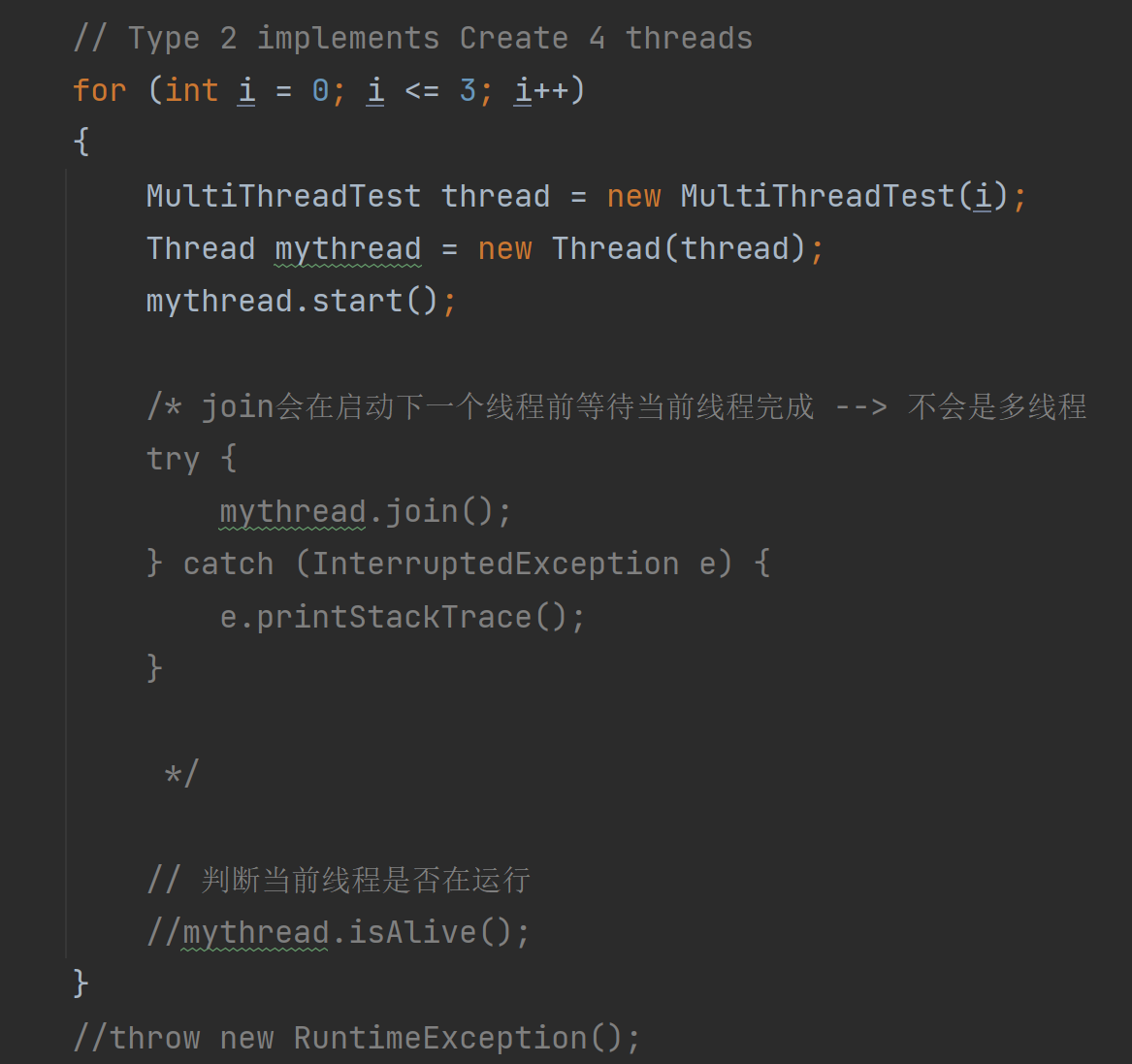
MultiThread.java
1 | public class MultiThreadTest implements Runnable |
Main.java
1 | for (int i = 0; i <= 3; i++) |
Differences & pros/cons between using extends or implements here
If we are using extends, which means the new class we create CAN NOT inherent from other classes anymore. Because in Java, we are not allow multi-inheritance which means each sub-class CAN ONLY HAVE ONE super class. If we are using extends then we have no chance to let that new class to inherent from other class.
But implements are different, a sub-class can only have one super class but it can have multiple interfaces. We can implements more than one interfaces if we like. By using implements Runnable to create a new thread we are still be able to extends that new class to some other super class.
Reference
About this Post
This post is written by Andy, licensed under CC BY-NC 4.0.